Cocoa Development
... an introduction
by Lieven Dekeyser
Part 1
- What is Cocoa?
- Objective-C
- C
- Foundation Framework
What is Cocoa
- Collection of frameworks
- Mac OS X: Foundation and AppKit
- iPhone OS: Foundation and UIKit
- Frameworks enable tools
- Interface builder (views)
- Interface builder (controllers)
- Xcode (code)
- Xcode (data models)
Cocoa on Mac OS X
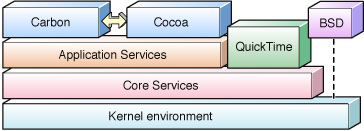
Cocoa on Mac OS X
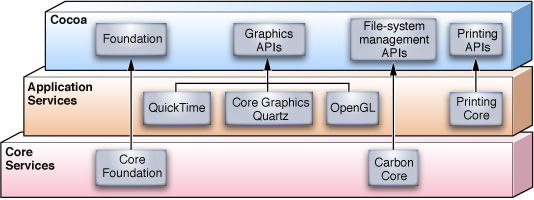
Cocoa on iPhone OS
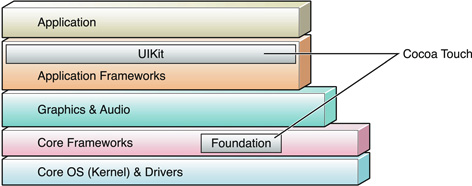
Objective-C
- Geschiedenis:
- Begin jaren '80: Brad Cox at StepStone
- 1988: gekozen door NeXTStep
- 1992: deel van de GCC collectie
- Heden: GNUStep, Mac OS X, iPhone OS, Cocotron, inspiratie voor Objective-J,...
- Net zoals C++ een objectgeoriënteerde uitbreiding op ANSI C, maar:
- Syntax geïnspireerd op SmallTalk
- Slechts 1 echte syntactische toevoeging aan C
- Dynamic typing and linking
C: Hello World
#include <stdio.h>
int main(int argc, const char * argv[])
{
printf("hello world");
return 0;
}
C: Hello World, explained
-
#include <stdio.h>
Tells the preprocessor to replace this line with the the contents of the file stdio.h
-
int main(int argc, const char * argv[])
int
: return type
main
: entry point of the application. Every C-application needs a main function
int argc
: first argument of main
, number of elements in argv
const char * argv[]
: array of elements of type const char *
C: Types
- int: Native integer format of the architecture
- char: Character, usually 1 byte
- float, double: Single and double precision floating point numbers
- enum: Enumeration
- struct: "aggregates a fixed set of labelled objects, possibly of different types, into a single object"
- union: "a datastructure that holds a value that may have any of several representations or formats"
C: Pointers
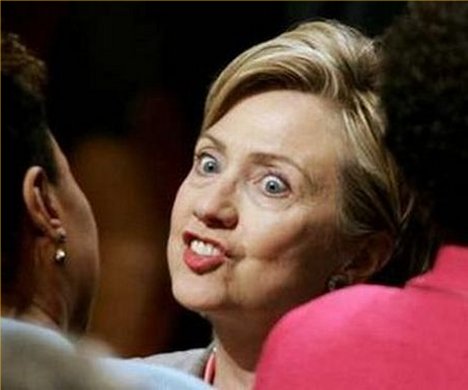
- Seriously, pointers aren't scary!
C: Pointers
- A pointer is just a variable that contains an address of a location in memory
- In other words, it points to that location
- Addresses are expressed as numbers. Size is architecture-dependent.
- Use the & operator to get the address of a variable
- Use the * operator to get the data a pointer points to (dereferencing)
C: Pointer gotchas
- Pointers allow you to manipulate any memory location in any way you'd want
- Dereferencing an invalid pointer can lead to crashes
- Stick to your own memory.
- Give pointers (but actually any variable) an initial value!
- Always reset pointers you don't need anymore to NULL
C: Strings
- There is no string type in C
- Just pointers to a location in memory which is interpreted as a 0-termintated block of characters.
- [address]->['a','b','c','\0'];
C: Memory allocation
- Variables are allocated on the stack.
- easy
- out of scope => deallocation
- limited in size (architecture-dependent)
- not flexible
- You can also allocate memory on the heap
- very flexible
- limited only by available (virtual) memory
- manual (de)allocation (malloc/free)
- requires discipline (who "owns" the memory?)
Objective-C
- C: basically a cross-architecture assembler with a nicer syntax
- You can write modern code in it though, see CoreFoundation
- Objective-C: let's get object-oriented!
Objective-C: Class interface
@interface Circle : NSObject
{
float mX;
float mY;
float mRadius;
}
- (id)init;
- (id)initWithRadius:(float)inRadius x:(float)inX y:(float)inY;
- (float)surface;
@end
Objective-C: Class implementation
@implementation Circle
- (id)init
{
return [self initWithRadius:0.0f x:0.0f y:0.0f];
}
- (id)initWithRadius:(float)inRadius x:(float)inX y:(float)inY
{
self = [super init];
if (self != nil)
{
mX = inX;
mY = inY;
mRadius = inRadius;
}
return self;
}
- (float)surface
{
return M_PI * mRadius * mRadius;
}
@end
Objective-C: Instances & messages
#import "Circle.h"
int main(int inNumArgs, const char * inArgs[])
{
Circle * circle = [[Circle alloc] initWithRadius:100.0f
x:10.0f y:5.0f];
float surface = [circle surface];
NSLog(@"Circle surface = %.2f", surface);
return 0;
}
Objective-C: Memory management
- All objects are allocated on the heap
- Allocation:
[ClassName alloc]
, followed by an init
message
- Deallocation:
[someObject release]
- Actually, that's not really true: Retain Count
- After alloc, retain count is 1
release
=> retainCount--
- When retain count == 0, object is deallocated
retain
=> retainCount++
Objective-C: Autorelease Pools
[someObject autorelease]
same as release but...
- ... if retain count == 0, put in autorelease pool in stead of being deallocated immediately
- Deallocated if autorelease pool is cleared
- Event-driven apps: every event-cycle
- In practice: ideal for temporary objects
- similar to variables on stack
The End
Questions?